Multithreading is a crucial concept in modern programming, allowing applications to perform multiple tasks simultaneously, thereby enhancing efficiency and responsiveness. In this article, we’ll delve into the realm of multithreading in PHP, exploring its implementation, best practices, challenges, and performance considerations. Although, PHP does not give inbuilt multi-threading functionality.
Table of Contents
Understanding Multithreading in PHP
What is Multithreading?
Multithreading refers to the concurrent execution of multiple threads within a single process. Each thread represents a separate flow of control, enabling tasks to be executed concurrently, leading to improved performance and resource utilization.
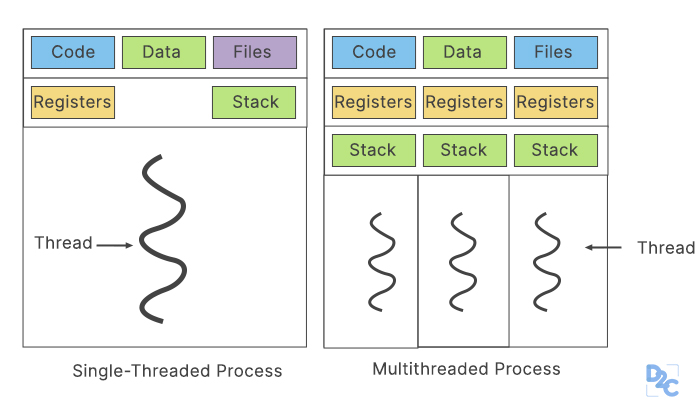
How Multithreading Works in PHP?
Traditionally, PHP has been criticized for its lack of native support for multithreading. However, with the introduction of the pthreads
extension, developers can now harness the power of multithreading in PHP. This extension provides a streamlined interface for creating and managing threads within PHP scripts.
Benefits of Multithreading in PHP
Multithreading in PHP offers several benefits, including:
- Improved performance through parallel execution of tasks.
- Enhanced responsiveness, particularly in I/O-bound applications.
- Efficient resource utilization, leading to better scalability.
Implementing Multithreading in PHP
Using pthreads
Extension
The pthreads
extension is a PHP extension that enables multithreading capabilities within PHP scripts. It provides classes and functions for creating and managing threads, as well as synchronization mechanisms for coordinating thread execution.
Here’s a simple example of creating and running multiple threads using pthreads
#!/usr/bin/php
<?php
class AsyncOperation extends Thread {
public function __construct($arg) {
$this->arg = $arg;
}
public function run() {
if ($this->arg) {
$sleep = mt_rand(1, 10);
printf('%s: %s - start - sleeps %d' . "\n", date("g:i:sa"), $this->arg, $sleep);
sleep($sleep);
printf('%s: %s - finish' . "\n", date("g:i:sa"), $this->arg);
}
}
}
// Create an array of threads
$stack = array();
foreach (range("A", "D") as $i) {
$stack[] = new AsyncOperation($i);
}
// Start the threads
foreach ($stack as $t) {
$t->start();
}
?>
Creating Threads in PHP
In PHP, threads are represented by instances of the Thread
class. To create a new thread, simply extend the Thread
class and implement the run
method, which contains the code to be executed by the thread.
Handling Synchronization
Synchronization is essential when dealing with shared resources in multithreaded applications. PHP provides built-in synchronization primitives such as mutexes, semaphores, and condition variables to facilitate thread-safe access to shared resources.
Best Practices for Multithreading in PHP
Thread Safety
Ensure that shared resources are accessed in a thread-safe manner to prevent data corruption and race conditions. Use synchronization primitives to enforce mutual exclusion and coordination between threads.
Resource Management
Proper resource management is crucial in multithreaded applications to prevent resource leaks and contention. Be mindful of resource allocation and deallocation within threads to avoid memory leaks and other resource-related issues.
Error Handling
Implement robust error-handling mechanisms to gracefully handle exceptions and errors that may arise during thread execution. Proper error handling can help prevent application crashes and ensure smooth operation.
Common Challenges and Solutions
Deadlocks and Race Conditions
Deadlocks and race conditions are common pitfalls in multithreaded programming. To mitigate these issues, use synchronization primitives judiciously and follow best practices for thread-safe programming.
Memory Leaks
Memory leaks can occur in multithreaded applications due to improper resource management. Monitor memory usage carefully and employ techniques such as reference counting and garbage collection to reclaim unused memory.
Debugging Multithreaded Applications
Debugging multithreaded applications can be challenging due to the complex interactions between threads. Use debugging tools and techniques such as logging and tracing to identify and resolve issues efficiently.
Performance Considerations
Scalability
Multithreading can improve scalability by allowing applications to handle multiple requests concurrently. However, excessive threading can lead to overhead and contention, so it’s essential to strike a balance between concurrency and resource consumption.
Overhead
Multithreading introduces overhead in terms of memory and CPU usage. Measure and optimize thread creation and management to minimize overhead and maximize performance.
Load Balancing
Effective load balancing is crucial for distributing workload evenly across threads and maximizing resource utilization. Implement load-balancing algorithms and strategies to ensure optimal performance in multithreaded applications.
Future of Multithreading in PHP
As PHP continues to evolve, we can expect further advancements in multithreading support and performance optimization. With the increasing demand for scalable and responsive web applications, multithreading will play a pivotal role in shaping the future of PHP development.
Conclusion
Multithreading in PHP opens up new possibilities for developing high-performance, scalable web applications. By understanding the principles of multithreading and following best practices, developers can harness the power of concurrency to build robust and efficient PHP applications.
Unique FAQs
- Can I use multithreading in all PHP applications?
- While multithreading can enhance performance, it may not be suitable for all PHP applications, especially those that are CPU-bound or have limited concurrency requirements.
- What are the limitations of multithreading in PHP?
- PHP’s multithreading capabilities are limited compared to languages like Java or C++. It’s essential to understand these limitations and use multithreading judiciously.
- How do I debug multithreaded PHP applications?
- Debugging multithreaded PHP applications can be challenging. Use tools like Xdebug or PHP’s built-in debugging features to trace and diagnose issues.
- What is the overhead associated with multithreading in PHP?
- Multithreading introduces overhead in terms of memory and CPU usage. It’s crucial to optimize thread creation and management to minimize this overhead.
- Is multithreading necessary for all PHP projects?
- Multithreading is not necessary for all PHP projects. It’s essential to assess the specific requirements of your application and determine whether multithreading would be beneficial.
References
[1] –multithreading – How can one use multi threading in PHP applications – Stack Overflow
[2] –Multithreading in PHP – Stack Overflow
[3] –How to Achieve Multithreading in PHP | Delft Stack
[4] –PHP and Threads: Multithreading and Parallel Execution in PHP | Reintech media
[5] –PHP: Introduction – Manual